expression_weighted (dCGP-W)
This class represents a Weighted Cartesian Genetic Program. Each node connection is associated to a weight so that more generic mathematical expressions can be represented. When instantiated with the type gdual<T>, also the weights are defined as gduals, hence the program output can be expanded also with respect to the weights thus allowing to train the weights using algorithms such as stochastic gradient descent, while the rest of the expression remains fixed.
The class template can be instantiated using the types double or gdual<T>.
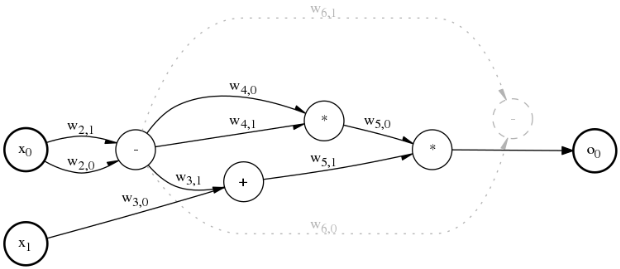
A weighted dCGP expression
-
template<typename T>
class expression_weighted : public dcgp::expression<T> A weighted dCGP expression.
This class represents a mathematical expression as encoded using CGP with the addition of weights on the connections. It contains algorithms to compute the value (numerical and symbolical) of the expression and its derivatives, as well as to mutate the expression.
- Template Parameters
T – expression type. Can be double, or a gdual type.
Public Functions
-
inline expression_weighted(unsigned n = 1u, unsigned m = 1u, unsigned r = 1u, unsigned c = 1u, unsigned l = 1u, unsigned arity = 2u, std::vector<kernel<T>> f = kernel_set<T>({"sum"})(), unsigned seed = dcgp::random_device::next())
Constructor.
Constructs a weighted dCGP expression.
- Parameters
n – [in] number of inputs (independent variables).
m – [in] number of outputs (dependent variables).
r – [in] number of rows of the cartesian representation of the expression as an acyclic graph.
c – [in] number of columns of the cartesian representation of the expression as an acyclic graph.
l – [in] number of levels-back allowed. This, essentially, controls the minimum number of allowed operations in the formula. If uncertain set it to c + 1
arity – [in] arities of the basis functions for each column.
f – [in] function set. An std::vector of dcgp::kernel<expression::type>.
seed – [in] seed for the random number generator (initial expression and mutations depend on this).
-
inline expression_weighted(unsigned n, unsigned m, unsigned r, unsigned c, unsigned l, std::vector<unsigned> arities, std::vector<kernel<T>> f, unsigned seed = dcgp::random_device::next())
Constructor.
Constructs a weighted dCGP expression
- Parameters
n – [in] number of inputs (independent variables).
m – [in] number of outputs (dependent variables).
r – [in] number of rows of the cartesian representation of the expression as an acyclic graph.
c – [in] number of columns of the cartesian representation of the expression as an acyclic graph.
l – [in] number of levels-back allowed. This, essentially, controls the minimum number of allowed operations in the formula. If uncertain set it to c + 1
arities – [in] arities of the basis functions (per each column).
f – [in] function set. An std::vector of dcgp::kernel<expression::type>.
seed – [in] seed for the random number generator (initial expression and mutations depend on this).
-
inline virtual std::vector<T> operator()(const std::vector<T> &in) const override
Evaluates the dCGP-weighted expression.
This evaluates the dCGP-weighted expression. This method overrides the base class method. NOTE we cannot template this and the following function as they are virtual in the base class.
- Parameters
in – [in] std::vector containing the values where the dCGP-weighted expression has to be computed
- Returns
The value of the output (an std::vector)
-
inline virtual std::vector<std::string> operator()(const std::vector<std::string> &in) const override
Evaluates the dCGP-weighted expression.
This evaluates the dCGP-weighted expression. This method overrides the base class method.
- Parameters
in – [in] an std::vector containing the symbol names.
- Returns
The symbolic value of the output (an std::vector)
-
template<typename U, functor_enabler<U> = 0>
inline std::vector<U> operator()(const std::initializer_list<U> &in) const Evaluates the dCGP expression.
This evaluates the dCGP expression. According to the template parameter it will compute the value (double) the Taylor expansion (gdual) or a symbolic representation (std::string). Any other type will result in a compilation-time error (SFINAE). This is identical to the other overload and is provided only for convenience
- Parameters
in – [in] an initializer list containing the values where the dCGP expression has to be computed (doubles, gduals or strings)
- Returns
The value of the function (an std::vector)
-
inline void set_weight(unsigned node_id, unsigned input_id, const T &w)
Sets a weight.
Sets a connection weight to a new value
- Parameters
node_id – [in] the id of the node whose weight is being set (convention adopted for node numbering http://ppsn2014.ijs.si/files/slides/ppsn2014-tutorial3-miller.pdf)
input_id – [in] the id of the node input (0 for the first one up to arity-1)
w – [in] the new value of the weight
- Throws
std::invalid_argument – if the node_id or input_id are not valid
-
inline void set_weights(const std::vector<T> &ws)
Sets all weights.
Sets all the connection weights at once
- Parameters
ws – [in] an std::vector containing all the weights to set
- Throws
std::invalid_argument – if the input vector dimension is not valid (r*c*arity)
-
inline T get_weight(unsigned node_id, unsigned input_id) const
Gets a weight.
Gets the value of a connection weight
- Parameters
node_id – [in] the id of the node (convention adopted for node numbering http://ppsn2014.ijs.si/files/slides/ppsn2014-tutorial3-miller.pdf)
input_id – [in] the id of the node input (0 for the first one up to arity-1)
- Throws
std::invalid_argument – if the node_id or input_id are not valid
- Returns
the value of the weight
Friends
-
inline friend std::ostream &operator<<(std::ostream &os, const expression_weighted &d)
Overloaded stream operator.
Will return a formatted string containing a human readable representation of the class
- Returns
std::string containing a human-readable representation of the problem.