expression_weighted (dCGP-W)
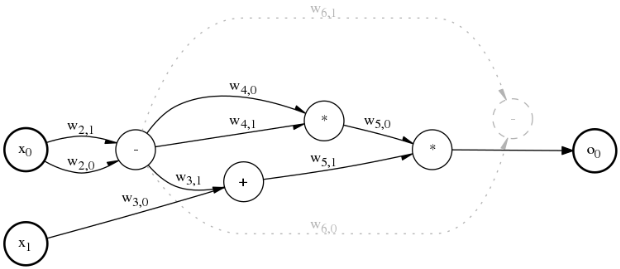
Three types of expression_weighted are currently available in python. They differ from the type they operate upon: double, gdual, vectorized gdual.
expression_weighted_double
- class dcgpy.expression_weighted_double(inputs, outputs, rows, cols, levels_back, arity = 2, kernels, n_eph = 0, seed = randint)
A weighted CGP expression
Constructs a CGP expression operating on double
- Parameters
inputs (
int
) – number of inputsoutputs (
int
) – number of outputsrows (
int
) – number of rows of the cartesian representation of the expression as an acyclic graph.cols (
int
) – number of columns of the cartesian representation of the expression as an acyclic graph.levels_back (
int
) – number of levels-back allowed. This, essentially, controls the minimum number of allowed operations in the formula. If uncertain set it to cols + 1arity (
int
onlist
) – arity of the kernels. Assumed equal for all columns unless its specified by a list. The list must contain a number of entries equal to the number of columns.kernels (
List[dcgpy.kernel_double]
) – kernel functionsn_eph (int) – Number of ephemeral constants. Their values and their symbols can be set via dedicated methods.
seed (
int
) – random seed to generate mutations and chromosomes
- eph_val
Values of the ephemeral constants.
- Type
list(
`)double`
- eph_symb
Symbols used for the ephemeral constants.
- Type
list(
str
)
Examples:
>>> from dcgpy import * >>> dcgp = expression_double(1,1,1,10,11,2,kernel_set_double(["sum","diff","mul","div"])(), 0, 32) >>> print(dcgp) ... >>> num_out = dcgp([in]) >>> sym_out = dcgp(["x"])
- get_weight(node_id, input_id)
Gets a weight.
Note
Convention adopted for node numbering: http://ppsn2014.ijs.si/files/slides/ppsn2014-tutorial3-miller.pdf
- Parameters
node_id (
int
) – the id of the nodeinput_id (
int
) – the id of the node input (0 for the first one up to arity-1)
- Returns
The value of the weight (a
float
)- Raises
ValueError – if node_id or input_id are not valid
- get_weights()
Gets all weights
- set_weight(node_id, input_id, weight)
Sets a weight.
Note
Convention adopted for node numbering: http://ppsn2014.ijs.si/files/slides/ppsn2014-tutorial3-miller.pdf
- Parameters
node_id (
int
) – the id of the node whose weight is being setinput_id (
int
) – the id of the node input (0 for the first one up to arity-1)weight (
float
) – the new value of the weight
- Raises
ValueError – if node_id or input_id are not valid
- set_weights(weights)
Sets all weights.
- Parameters
weights (
List[float]
) – the new values of the weights- Raises
ValueError – if the input vector dimension is not valid (r*c*arity)
expression_weighted_gdual_double
- class dcgpy.expression_weighted_gdual_double(inputs, outputs, rows, cols, levels_back, arity = 2, kernels, n_eph = 0, seed = randint)
A weighted CGP expression
Constructs a CGP expression operating on gdual_double
- Parameters
inputs (
int
) – number of inputsoutputs (
int
) – number of outputsrows (
int
) – number of rows of the cartesian representation of the expression as an acyclic graph.cols (
int
) – number of columns of the cartesian representation of the expression as an acyclic graph.levels_back (
int
) – number of levels-back allowed. This, essentially, controls the minimum number of allowed operations in the formula. If uncertain set it to cols + 1arity (
int
onlist
) – arity of the kernels. Assumed equal for all columns unless its specified by a list. The list must contain a number of entries equal to the number of columns.kernels (
List[dcgpy.kernel_gdual_double]
) – kernel functionsn_eph (int) – Number of ephemeral constants. Their values and their symbols can be set via dedicated methods.
seed (
int
) – random seed to generate mutations and chromosomes
- eph_val
Values of the ephemeral constants.
- Type
list(
`)gdual_double`
- eph_symb
Symbols used for the ephemeral constants.
- Type
list(
str
)
Examples:
>>> from dcgpy import * >>> dcgp = expression_gdual_double(1,1,1,10,11,2,kernel_set_gdual_double(["sum","diff","mul","div"])(), 0, 32) >>> print(dcgp) ... >>> num_out = dcgp([in]) >>> sym_out = dcgp(["x"])
- get_weight(node_id, input_id)
Gets a weight.
Note
Convention adopted for node numbering: http://ppsn2014.ijs.si/files/slides/ppsn2014-tutorial3-miller.pdf
- Parameters
node_id (
int
) – the id of the nodeinput_id (
int
) – the id of the node input (0 for the first one up to arity-1)
- Returns
The value of the weight (a
float
)- Raises
ValueError – if node_id or input_id are not valid
- get_weights()
Gets all weights
- set_weight(node_id, input_id, weight)
Sets a weight.
Note
Convention adopted for node numbering: http://ppsn2014.ijs.si/files/slides/ppsn2014-tutorial3-miller.pdf
- Parameters
node_id (
int
) – the id of the node whose weight is being setinput_id (
int
) – the id of the node input (0 for the first one up to arity-1)weight (
float
) – the new value of the weight
- Raises
ValueError – if node_id or input_id are not valid
- set_weights(weights)
Sets all weights.
- Parameters
weights (
List[float]
) – the new values of the weights- Raises
ValueError – if the input vector dimension is not valid (r*c*arity)
expression_weighted_gdual_vdouble
- class dcgpy.expression_weighted_gdual_vdouble(inputs, outputs, rows, cols, levels_back, arity = 2, kernels, n_eph = 0, seed = randint)
A weighted CGP expression
Constructs a CGP expression operating on gdual_vdouble
- Parameters
inputs (
int
) – number of inputsoutputs (
int
) – number of outputsrows (
int
) – number of rows of the cartesian representation of the expression as an acyclic graph.cols (
int
) – number of columns of the cartesian representation of the expression as an acyclic graph.levels_back (
int
) – number of levels-back allowed. This, essentially, controls the minimum number of allowed operations in the formula. If uncertain set it to cols + 1arity (
int
onlist
) – arity of the kernels. Assumed equal for all columns unless its specified by a list. The list must contain a number of entries equal to the number of columns.kernels (
List[dcgpy.kernel_gdual_vdouble]
) – kernel functionsn_eph (int) – Number of ephemeral constants. Their values and their symbols can be set via dedicated methods.
seed (
int
) – random seed to generate mutations and chromosomes
- eph_val
Values of the ephemeral constants.
- Type
list(
`)gdual_vdouble`
- eph_symb
Symbols used for the ephemeral constants.
- Type
list(
str
)
Examples:
>>> from dcgpy import * >>> dcgp = expression_gdual_vdouble(1,1,1,10,11,2,kernel_set_gdual_vdouble(["sum","diff","mul","div"])(), 0, 32) >>> print(dcgp) ... >>> num_out = dcgp([in]) >>> sym_out = dcgp(["x"])
- get_weight(node_id, input_id)
Gets a weight.
Note
Convention adopted for node numbering: http://ppsn2014.ijs.si/files/slides/ppsn2014-tutorial3-miller.pdf
- Parameters
node_id (
int
) – the id of the nodeinput_id (
int
) – the id of the node input (0 for the first one up to arity-1)
- Returns
The value of the weight (a
float
)- Raises
ValueError – if node_id or input_id are not valid
- get_weights()
Gets all weights
- set_weight(node_id, input_id, weight)
Sets a weight.
Note
Convention adopted for node numbering: http://ppsn2014.ijs.si/files/slides/ppsn2014-tutorial3-miller.pdf
- Parameters
node_id (
int
) – the id of the node whose weight is being setinput_id (
int
) – the id of the node input (0 for the first one up to arity-1)weight (
float
) – the new value of the weight
- Raises
ValueError – if node_id or input_id are not valid
- set_weights(weights)
Sets all weights.
- Parameters
weights (
List[float]
) – the new values of the weights- Raises
ValueError – if the input vector dimension is not valid (r*c*arity)